In this blog, we will be learning how to draw the Facebook logo using the Python programming language and the turtle graphics library. The turtle graphics library is a popular tool for introducing kids to programming and for creating simple animations.
In this project, I used pycharm IDE. How to install pycharm in windows: https://iterathon.tech//how-to-install-the-pycharm-in-windows/
Turtle Library
To get started, you will need to install the turtle library in your Python environment. If you already have Python installed, you can install the turtle library by running the following command in your terminal or command prompt:
pip install turtle
Link to know about turtle graphics: https://docs.python.org/3/library/turtle.html
Import Turtle
Now that we have the turtle library installed, let’s start by importing it in our Python script and setting up the screen:
from turtle import *
Let’s Start
The screen.bgcolor
function sets the background color of the turtle screen to blue, which is the main color used in the Facebook logo.Next, let’s create our turtle object and set its shape, color, and speed:
speed(10) color("#0270d6") Screen().bgcolor('black')
Now that we have our turtle set up, let’s start drawing the Facebook logo. The logo consists of a white rectangle and a white lowercase letter “f” inside of it. Let’s start by drawing the square:
#setup the coordinates penup() goto(0, 150) pendown() #Draw the rectangle begin_fill() forward(150) circle(-50, 90) forward(300) circle(-50, 90) forward(300) circle(-50, 90) forward(300) circle(-50, 90) forward(150) end_fill()
The above code uses a for loop to draw the square. It moves the turtle to the starting point using the goto
function and then uses the pendown
function to start drawing. The loop then draws each of the four sides of the rectangle using the forward
function and the right
function to turn the turtle at each corner.
Draw F
#drawing the letter F color("white") penup() goto(140, 80) pendown()
The above code first moves the turtle to the starting point for the letter “f” and then uses a background colour of white.
Full Code
from turtle import * speed(10) color("#0270d6") Screen().bgcolor('black') #setup the coordinates penup() goto(0, 150) pendown() #Draw the rectangle begin_fill() forward(150) circle(-50, 90) forward(300) circle(-50, 90) forward(300) circle(-50, 90) forward(300) circle(-50, 90) forward(150) end_fill() #drawing the letter F color("white") penup() goto(140, 80) pendown() begin_fill() right(180) forward(50) circle(80, 90) forward(50) right(90) forward(80) left(90) forward(40) left(90) forward(80) right(90) forward(160) left(90) forward(55) left(90) forward(160) right(90) forward(70) left(80) forward(45) left(100) forward(80) right(90) forward(40) circle(-40, 90) forward(40) left(90) forward(45) end_fill() hideturtle() done()
Output
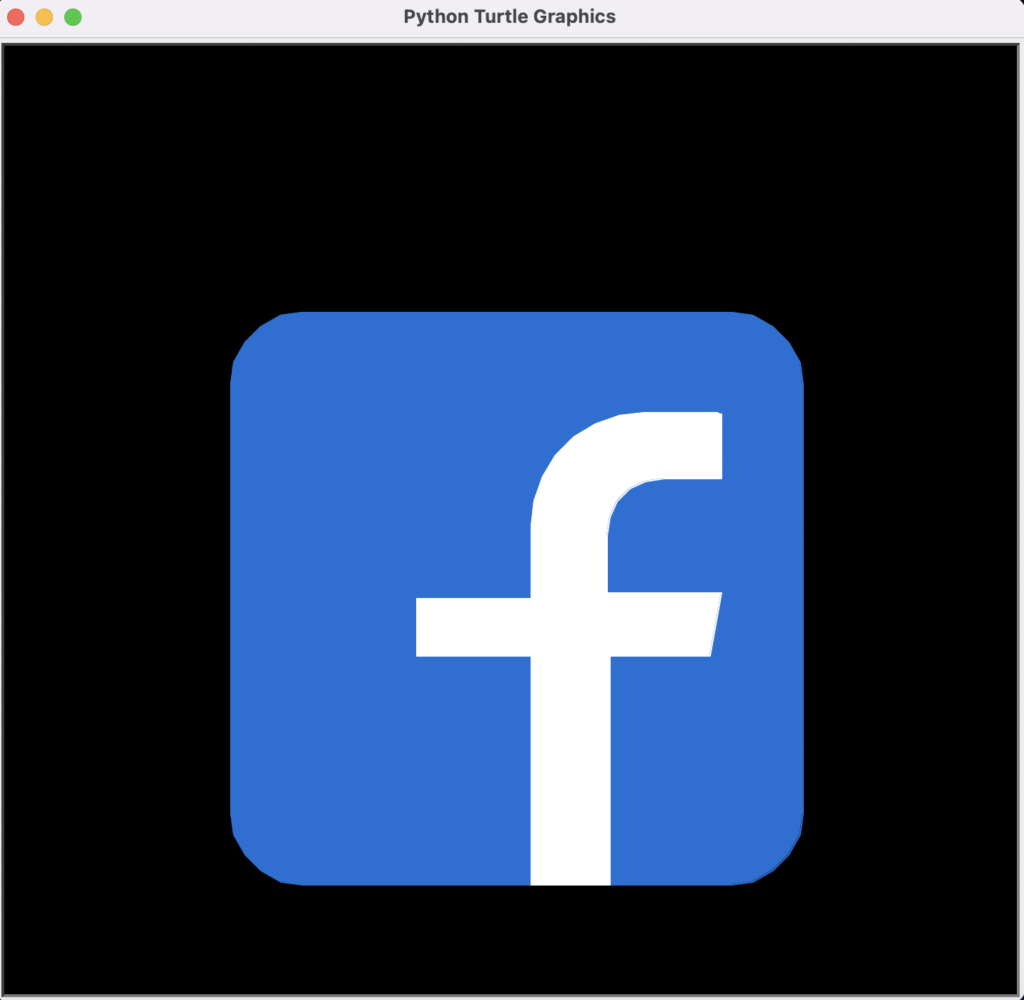
Save File
To run the script, simply save it to a file with a .py
extension and run it using the following command:
python filename.py
And there you have it, a simple program to draw the Facebook logo using the turtle graphics library in Python. This example demonstrates how easy it is to create simple graphics using turtle and provides a starting point for further exploration and experimentation with the library.
In conclusion, the turtle graphics library is a fun and accessible way to get started with programming and graphics in Python. By following the steps outlined in this blog, you can easily create your own graphics and animations using turtle. Additionally, the turtle library can be used as a platform for exploring more complex concepts in computer graphics, such as creating animations, creating complex shapes, and working with colors and images.
So why not give it a try? Start experimenting with turtle today, and see where your creativity takes you!
Related Post
Implementing Transformer Models in Python: https://iterathon.tech//a-beginners-guide-to-implementing-transformer-models-in-python/
Random Password Generator in Python: https://iterathon.tech//random-password-generator-in-python/
Python for Data Analysis and Predictive Modelling: https://iterathon.tech//discovering-the-magic-of-python-for-data-analysis-and-predictive-modeling/
LEARN SOMETHING NEW ❤️