python functions and their types
Function Definition
- A function is a group of statements that perform a specific task (or)
- A function is an organized block of code that performs to do some tasks.
Main Advantages of Functions
- It avoids repetition and makes a high degree of code reusing.
- It provides better modularity for your application.
Define Function
Before we create a function, we want to know some basic points to define a function.
- Functions begin with the keyword “def ” followed by function name and parenthesis ().
- The body of the statements always comes after a colon (:) and is indented.
- Finally calling a Function with function name and ().
The syntax for defining Function:
def function_name(parameter1, parameter2, ...): <Body of the statement> function_name()
Parameters, Arguments, and return are not compulsory ones but when needed, you can use them.
Note: Python keywords should not be used as the function names.
Calling a Function
# Example program def blog(): print("Iterathon") blog() #Output Iterathon
When you call the “blog” function with the blog name and parenthesis, the program displays the “Iterathon” as output.
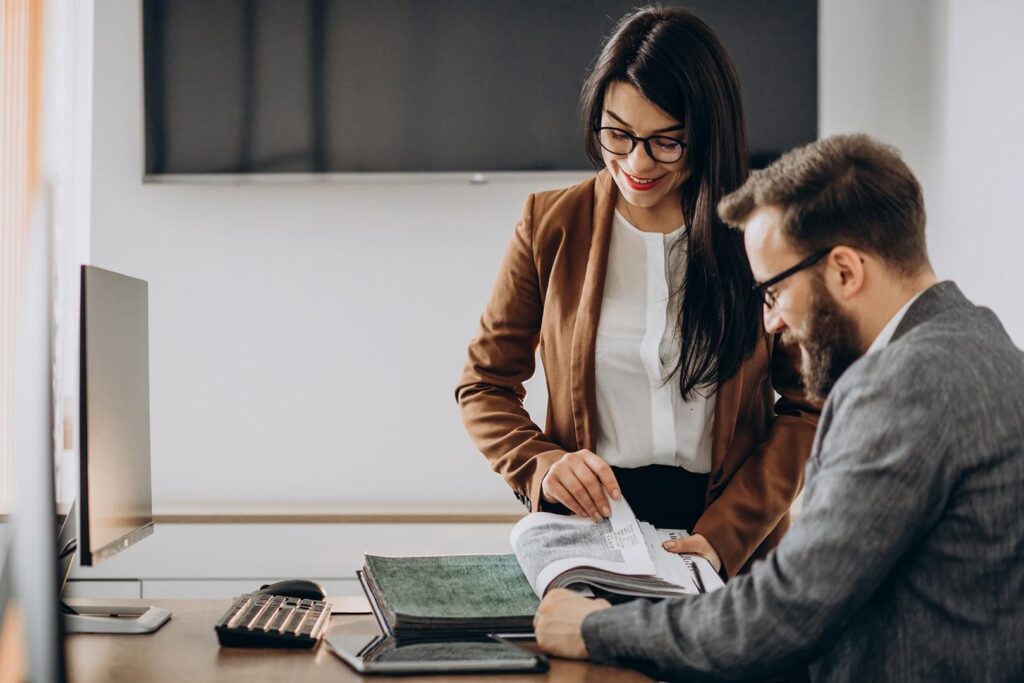
Function Types
Basically, Python functions are divided into the following ways
- User-defined Functions
- Bulit in Fuctions
- Lambda Functions
- Recursive Functions
User-defined Functions
Functions are defined by the user themselves. The user gives any function name but not a keyword as the function name.
#ExampleProgram def add(a,b): c = a+b print(c) add(5,7) #Output 12
The above example, add() is user defined function created and passed two arguments as 5 and 7. That 5, 7 are stored in a, b and these variables undergoes addition and gives a output as 12.
Built in Functions
Functions that are already organised, compiled and stored in python library.
Some Examples for Built in functions :
- print()
- input()
- sum()
- format()
- id()
Built-in Mathematical Functions in python: https://iterathon.tech//built-in-mathematical-functions-in-python/
Lambda Functions
In Python, Lambda function is a function that is defined with a “lambda” keyword. while other function are defined with “def” keyword.
Lambda functions are also called as Anonymous functions or unnamed functions.
#Lambda Function Example add = lambda a, b: a + b print ('The Sum is :', add(5,7)) #Output The Sum is : 12
Recursive Functions
When a function calls itself is known as recursion. Recursion works like loop but sometimes it makes more sense to use recursion than loop.
#Recursive Function
def fact(n):
if n == 0:
return 1
else:
return n * fact (n-1)
print (fact (5))
Previous Blog
10 Awesome Python Tips and Tricks You Should Learn today : https://iterathon.tech//10-awesome-python-tips-and-tricks-you-should-learn/
LEARN MORE ❣