Python has experienced impressive growth as compared to the other language. so here are the tips and tricks that help to improve the performance of your Python programming and solving ability. Here are the 10 awesome python tips and tricks you must learn today.
1. Fulfilled all conditions without declared all conditions in if.
# For one or two conditions
viewers = 1000
likes = 500
if viewers >= 1000 and likes >= 500:
print(" Good luck ")
#Output
Good luck
If you have one or two conditions, you can use the above example programs
# For more than ten conditions
viewers = 2000
likes = 700
conditions = [ viewers >= 1000,likes >= 500]
if all(conditions):
print(" Good luck ")
#Output
Good luck
Suppose if you have more than ten conditions, you can create a list and use all() to perform AND operation (fulfilled all conditions). If you didn’t get it compare two examples side by side to understand clearly.
2. Fulfilled at least one conditions without declared all conditions in if.
# For one or two conditions
viewers = 1000
likes = 500
if viewers >= 1000 or likes >= 700:
print(" Good luck ")
#Output
Good luck
# For more than ten conditions
viewers = 2000
likes = 700
conditions = [ viewers >= 1000,likes >= 500]
if any(conditions):
print(" Good luck ")
#Output
Good luck
You can create a list and use any() to perform OR operation (fulfilled at least one condition). If you didn’t get it compare two examples side by side to understand clearly.
3. Swapping two values without using temp
# Swapping two values
a,b = 5,7
# Swapping without using temp variable
a,b = b,a
print('a =' , a ,'b =' , b)
#Output
a = 7 b = 5
4. Remove Duplication in list ( Easy Way )
#Remove duplicates in List
Org_list = [1,2,3,4,2,3,5]
alter_list = list(set(Org_list))
print(alter_list)
#Output
[1, 2, 3, 4, 5]
We already know that set returns the values without duplicates. So, we convert a list to set and again set to list (Typecasting).
5. Reverse String
# Simple way to reverse string
Blog = "Iterathon" [::-1]
print(Blog)
#Output
nohtaretI
We have a lot of ways to reverse a string using python. But, most of the people didn’t know this.
6. Find The Most Frequent Value In a List
#Find most frequent value in a list
list = [2,4,6,2,5,3,2,1,7]
print(max(set(list), key = list.count))
#Output
2
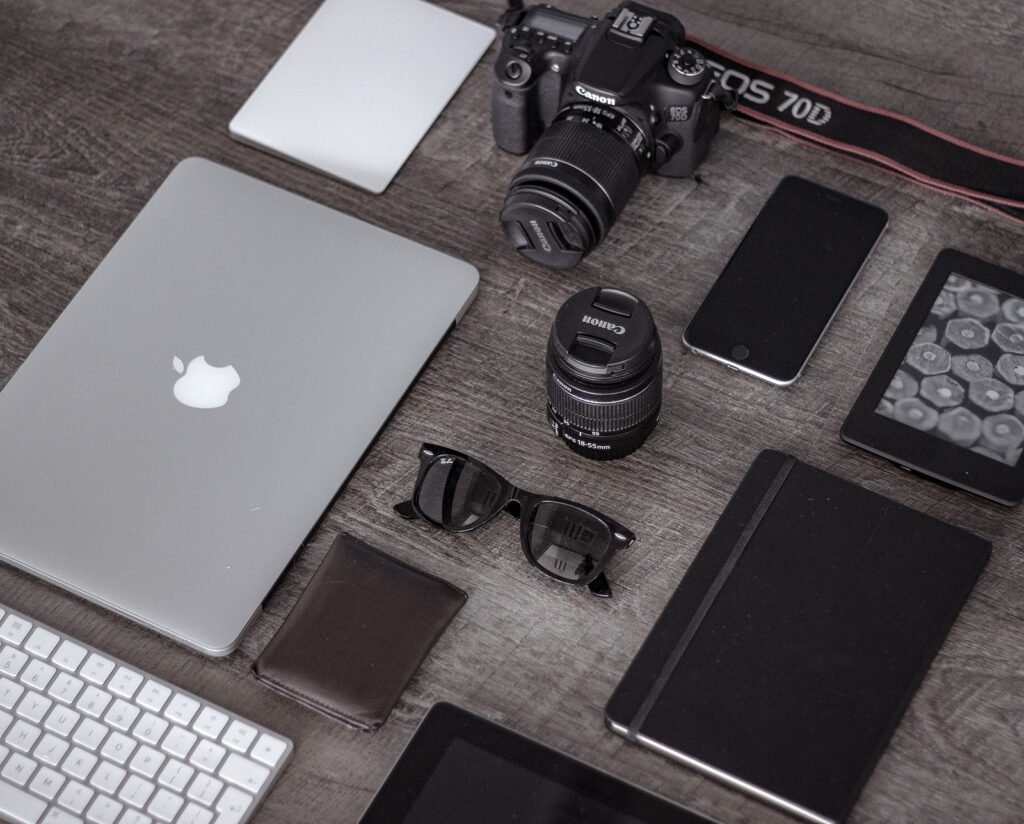
7. Generating squares of first 10 natural numbers (using comprehensions )
# Normal format
squares = [ ]
for x in range(1,11):
s = x ** 2
squares.append(s)
print (squares)
#Output
[1, 4, 9, 16, 25, 36, 49, 64, 81, 100]
List comprehension is the simplest way of creating a sequence of elements that fulfilled the condition
#List comprehension
squares = [ x ** 2 for x in range(1,11) ]
print (squares)
#Output
[1, 4, 9, 16, 25, 36, 49, 64, 81, 100]
8. Concatenating Strings
#join strings
characters = ['I', 'T', 'E', 'R', 'A', 'T', 'H', 'O', 'N']
Blog = ''.join(characters)
print(Blog)
#Output
ITERATHON
9. Colored Text using Python
Before doing this, you must have termcolor module to access this example. Link for Download termcolor: https://pypi.org/project/termcolor/
#Text with colors
from termcolor import colored
print(colored("ITERATHON", "red"))
#output
ITERATHON
10. Measuring Execution Time using Python
time module is an inbuilt module so you can access it without installing any extra packages or modules
#calculate Execution time
import time
start = time.time()
end = time.time()
total = end - start
print("Total Execuation time is", total)
#Output
Total Execuation time is 2.384185791015625e-07
Previous Blogs
Various built in functions to manipulate strings: https://iterathon.tech//various-built-in-functions-in-python/
LEARN LITTLE BY LITTLE TO CHANGE THE TECH WORLD