An array is a linear data structure that stores values of the same data type. To use arrays in python language, you need to import the standard array module.
The array is not a fundamental data type like strings, integers, etc. so we need to import the array module in python.
Arrays
#Syntax for import array from array import *
Once you have imported the array module, you can declare an array.
#Syntax for declaring array arrayName = array(typecode, [Initializers])
- array_name –> name of array
- typecode –> the type of array
- Initializers –> values
Example
#Example for an array from array import * my_array = array('i', [1,2,3,4,5]) for i in my_array: print(i) #Output 1 2 3 4 5
Here is a simple example of an array containing 5 integers.
The main difference between arrays and lists
In python, lists can contain values corresponding to different data types but arrays in python can only contain values corresponding to same data type
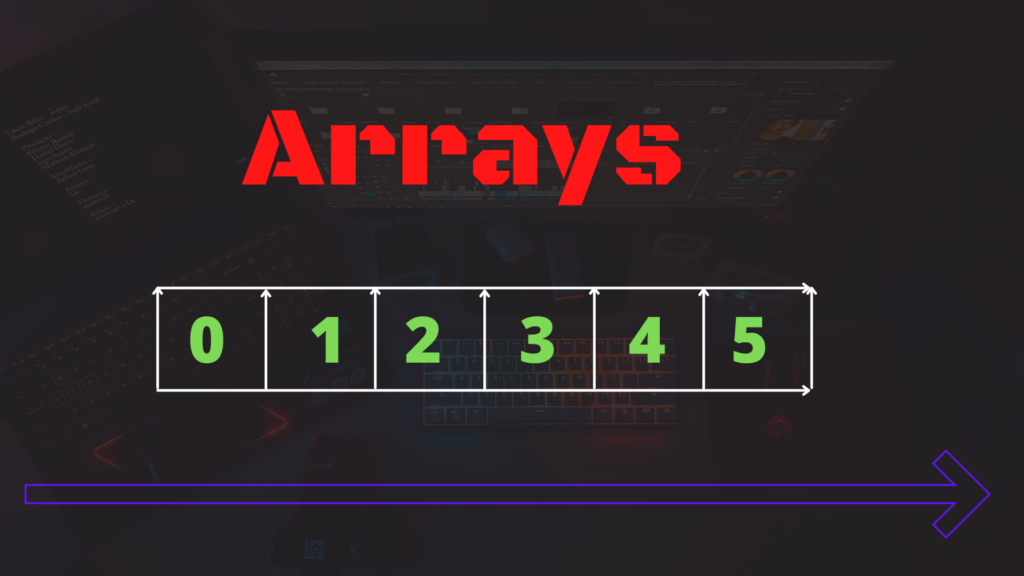
append values
The append() function is used to adds a value at the end of an array.
#append value 6 at the end of an array from array import * my_array = array('i', [1,2,3,4,5]) my_array.append(6) print(my_array) #Output array('i', [1, 2, 3, 4, 5, 6])
insert values
The insert() function inserts a value at the specified index in an array.
#append value 6 at the end of an array from array import * my_array = array('i', [1,2,3,4,5]) my_array.insert(0,0) print(my_array) #Output array('i', [0,1, 2, 3, 4, 5])
extend values
extend() is used to adds the elements in a list to the end of another list. That is concatenated (+ or +=) both lists.
#extend an array from array import * my_array = array('i', [1,2,3,4,5]) my_array1 = array('i', [6,7,8,9,10]) my_array.extend(my_array1) print(my_array) #Output array('i', [1, 2, 3, 4, 5, 6, 7, 8, 9, 10])
Add items using fromlist() method
Add items from list into array using fromlist() method
#extend an array from array import * my_array = array('i', [1,2,3,4,5]) array = [6,7,8] my_array.fromlist(array) print(my_array) #Output array('i', [1, 2, 3, 4, 5, 6, 7, 8])
Read also
10 Python shortcuts you need to know: https://cybrblog.tech/10-python-shortcuts-you-need-to-know/
Previous Blog
How to Display Alert Message using Python: https://iterathon.tech//how-to-display-alert-message-using-python/
LEARN SOMETHING NEW ❣