Frozenset Vs Set in python with Examples
Now, we are back with the awesome topic of advanced data type named frozen set. we are already seen that what is datatype and its types. Now, we are lightly moving with the frozen set data types. Before we learn advanced data types, let’s revise a bit about data types and their types.
Important datatypes: https://iterathon.tech//fundamentals-of-python-programming-with-examples/
Following are the data types that we have seen in previous blogs. Let’s revise them quickly.
- List
- Tuple
- Set
- Dictionary
- String
- Numbers
- Boolean
Set
Set in python is a data structure or data type that allows storing lots of mutable data into a single variable. The elements of the sets are unordered, there is no index number associated with them. Therefore, accessing the set elements with the help of index numbers is not possible.
Set are mutable just like a list which means, once a set is defined we can modify and update it later. we already know that set returns an element without duplicate.
Accessing and Manipulating Set
We know that there are various operations on perform using set and also manipulate them using various built-in functions. Let’s see a clever example. Link for download python interpreter for more practice: https://www.python.org/downloads/
#Accessing Elements through for loop
Blog = {"hello", "Iterathon", "I", "am", "There"}
for i in Blog:
print(i)
#Output
There
Iterathon
I
am
hello
Manipulating using some built-in functions
Set is a most basic level datatype, It supports all the method operations of the set such as add(), remove(), and so on.
Blog = {"Java", "C++", "C", "Python"}
Blog.add("Ruby")
print(Blog)
Blog.discard("C++")
print(Blog)
Blog.remove("C")
print(Blog)
#Output
{'C++', 'Python', 'C', 'Java', 'Ruby'}
{'Python', 'C', 'Java', 'Ruby'}
{'Python', 'Java', 'Ruby'}
In the example, I used two built-in functions discard and remove. Now, we will see what’s the difference between them.
Python provides the remove() and discard() method which helps us to remove the required element from a set by passing it as an argument.
Both work exactly the same, only the major difference is that, if the element to be deleted from the set using discard() doesn’t exist in the set, Python will not give the error. The program maintains its control flow.
While, if the element to be deleted from the set using remove() doesn’t exist in the set, Python will raise an error.
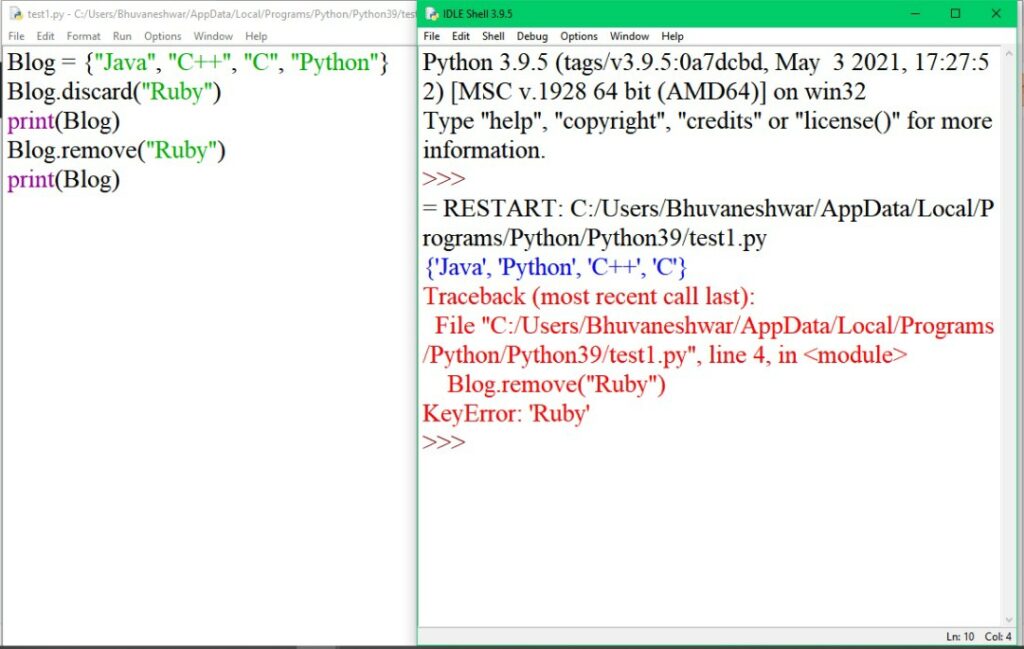
Frozen Set
We already saw that set data type. Now, we newly discussed the friend of set data types named frozen set. Frozen means unmoving or fixed.
The frozenset() is an inbuilt function in python that takes an iterable object as input and makes them immutable. It simply freezes the iterable objects and makes them unchangeable.
Frozenset is a new class that has the characteristics of a set, but its elements cannot be changed once assigned. That is once you created the set, it becomes immutable. Frozenset is also called a read-only set.
Frozenset Vs Set
Set is a most basic level datatype, It supports all the method operations of the set such as add(), remove(), and so on.
A = {1,2,3,4}
# Perform add() operation
A.add(8)
print(A)
#Output
>>>{1,2,3,4,8}
The Frozen set is immutable, it does not support any operations like add(), remove(), and so on.
A = frozenset([1,2,3,4])
# Perform add() operation
A.add(8)
print(A)
#output
Traceback (most recent call last):
File "<string>", line 9, in <module>
AttributeError: 'frozenset' object has no attribute 'add'
My Previous Post
Important interview questions in python: https://iterathon.tech//important-interview-questions-in-python/
LEARN LITTLE BY LITTLE TO CHANGE THE TECH WORLD